हेल्लो दोस्तों स्वागत है आपका हमारी एक और C Programming Language कि फायदेमंद पोस्ट में | इस पोस्ट में C Language Program Notes यानि कि सी लैंग्वेज के सभी प्रोग्राम के नोट्स को बोहोत ही आसान तरीके से इस पोस्ट में दर्शाया गया है |
यहाँ पर कुछ जरुरी बेसिक C programs को उनके इनपुट और आउटपुट के साथ में दर्शाया गया है जिन्हें समझना बोहोत ही आसान है और इन सभी c programs के codes को आप बोहोत ही आसानी से right side में ऊपर की ओर दिए गए copy button पर क्लिक करके कॉपी करके अपने अभ्यास के लिए और समझने के लिए प्रयोग भी कर सकते है |
Ques 1. Write a program to add three numbers.
#include<stdio.h> main() { int a, b, c, s; scanf("%d%d%d", &a, &b, &c); s = a + b + c; printf("sum=%d", s); }
OUTPUT
Enter three numbers = 1 2 3
Sum of three numbers = 6
Ques 2. Write a program to find average of five integer numbers.
#include<stdio.h> main() { int a,b,c,d,e; float avg; printf("Enter five integer numbers = "); scanf("%d%d%d%d%d",&a,&b,&c,&d,&e); avg = (a+b+c+d+e)/5.0; printf("Average of five integer numbers = %f",avg); }
OUTPUT
Enter five integer numbers = 1 2 3 4 5
Average of five integer numbers = 3.000000
Ques 3. Write a program to find area of circle. Radius of circle is given by user.
#include<stdio.h> main() { float r,area; // r= Radius of circle printf("Enter radius of circle = "); scanf("%f",&r); area = 3.14*r*r; // Value of Pie = 3.14 printf("Area of circle = %f",area); }
OUTPUT
Enter radius of circle = 5
Area of circle = 78.500000
Ques 4. Write a program to find area and perimeter of rectangle.
#include <stdio.h> main() { int a, b, Area, Perimeter; printf("Enter value of a = "); scanf("%d", &a); printf("Enter value of b = "); scanf("%d", &b); Area = (a * b); // Area of Rectangle = a * b printf("Area of rectangle = %d\n", Area); Perimeter = 2 * (a + b); // Perimeter of a Rectangle = 2(a+b) printf("Perimeter of rectangle = %d", Perimeter); }
OUTPUT
Enter value of a = 4
Enter value of b = 5
Area of rectangle = 20
Perimeter of rectangle = 18
Ques 5. Write a program to find simple interest and compound interest for given amount , rate of interest and time period.
#include <stdio.h> #include <math.h> main() { float p, r, t, SI, CI; printf("Enter Principle = "); scanf("%f", &p); printf("Enter Rate = "); scanf("%f", &r); printf("Enter Time = "); scanf("%f", &t); SI = (p * r * t) / 100; // Formula of Simple Interest printf("Simple Interest = %f\n", SI); CI = p * pow((1 + r / 100), t); // Formula of Compound Interest printf("Compound Interest = %f\n", CI); }
OUTPUT
Enter Principle = 5
Enter Rate = 4
Enter Time = 3
Simple Interest = 0.600000
Compound Interest = 5.624320
Ques 6. Write a program to convert the given temperature from Celsius to Fahrenheit.
#include <stdio.h> main() { float c, f; // c= Temperature in Celsius , f=Temperature in fahrenheit printf("Enter Temperature in Celsius = "); scanf("%f", &c); // Formula of Temperature Convert Celsius To Fahrenheit f = (c * 9) / 5 + 32; // c = (f-32)*5/9 printf("Temperature in Fahrenheit = %f", f); }
OUTPUT
Enter Temperature in Celsius = 5
Temperature in Fahrenheit = 41.000000
Ques 7. Distance in meter is given by user. Write a program to convert the given distance in kilometer and centimeter.
#include <stdio.h> main() { float cm, m, km; //cm = Centimeter , m = Meter , km = Kilometer printf("Enter Distance in Meter = "); scanf("%f", &m); km = m / 1000; printf("Distance Meter to Kilometer = %f\n", km); cm = m * 100; printf("Distance Meter to Centimeter = %f", cm); }
OUTPUT
Enter Distance in Meter = 5
Distance Meter to Kilometer = 0.005000
Distance Meter to Centimeter = 500.000000
Ques 8. Three sides of a triangle are given by the user. Write a program to find area and perimeter of triangle.
#include <stdio.h> #include <math.h> main() { float a, b, c, s, A, P; // Triangle three sides = a,b,c ,Area = A,Perimeter = P,Semiperimeter = s printf("Enter three sides of triangle = "); scanf("%f%f%f", &a, &b, &c); P = (a + b + c); printf("Perimeter of triangle = %f\n", P); s = (a + b + c) / 2; printf("Semi-Perimeter of triangle = %f\n", s); A = sqrt(s * (s - a) * (s - b) * (s - c)); printf("Area of triangle = %f", A); }
OUTPUT
Enter three sides of triangle = 5 8 11
Perimeter of triangle = 24.000000
Semi-Perimeter of triangle = 12.000000
Area of triangle = 18.330303
Ques 9. Write a program to check weather given number is positive or negative.
#include <stdio.h> main() { int a; printf("Enter number = "); scanf("%d", &a); a > 0 ? printf("This number is positive") : printf("This number is negative"); }
OUTPUT
Enter number = 10
This number is positive
// OR
Enter number = -20
This number is negative
Ques 10. Write a program to check weather given number is even or odd.
#include<stdio.h> main() { int a; printf("Enter number = "); scanf("%d",&a); a%2==0?printf("Number is odd"):printf("Number is even"); }
OUTPUT
Enter number = 11
Number is even
// OR
Enter number = 16
Number is odd
Ques 11. Write a program to check weather given year is leap or not.
#include<stdio.h>
main()
{
int a;
printf("Enter year = ");
scanf("%d",&a);
a%4==0?printf("This year is Leap"):printf("This year is not Leap");
}
Enter year = 2016
This year is Leap
// OR
Enter year = 2021
This year is not Leap
Ques 12. Write a program to check weather given number is positive , negative or zero.
#include <stdio.h>
main()
{
int a;
printf("Enter Number = ");
scanf("%d", &a);
a > 0 ? printf("%d is Positive", a) : (a < 0 ? printf("%d is Negavite", a) : printf("Zero"));
}
Enter Number = 25
25 is Positive
// OR
Enter Number = -12
-12 is Negavite
// OR
Enter Number = 0
Zero
Ques 13. Write a program to check weather given year is century leap or not.
#include <stdio.h>
main()
{
int a;
printf("Enter Year = ");
scanf("%d", a);
a % 100 == 0 ? (a % 400 == 0 ? printf("Century Leap Year") : printf("Not Century Leap Year")) : (a % 4 == 0 ? printf("Century Leap Year") : printf("Not Century leap year"));
}
Enter Year = 2023
Century Leap Year
Ques 14. Write a program to find greater of given two numbers using conditional operator.
#include <stdio.h>
main()
{
int a, b;
printf("Enter two numbers = ");
scanf("%d%d", &a, &b);
a > b ? printf("%d is Greater", a) : printf("%d is Greater", b);
}
Enter two numbers = 5 9
9 is Greater
Ques 15. Write a program to find smallest of given three numbers using conditional operators.
#include <stdio.h>
main()
{
int a, b, c;
printf("Enter three numbers = ");
scanf("%d%d%d", &a, &b, &c);
a < b ? printf("%d is Smallest", a) : (b < c ? printf("%d is Smallest", b) : printf("%d is Smallest", c));
}
Enter Three numbers = 66 12 42
Smallest Numbers : 12
Ques 16. Write a program to check weather a given point ( x , y ) lies on x-axis , y-axis or origin using conditional operators.
#include <stdio.h>
main()
{
int x, y;
printf("Enter points x, y = ");
scanf("%d%d", &x, &y);
x==0&&y==0 ? printf("Origin") : x==0&&y>0?printf("Y-Axis"): x>0&&y==0?printf("X-Axis"):printf("None");
}
Enter points x, y = 1 0
X-Axis
// OR
Enter points x, y = 0 1
Y-Axis
// OR
Enter points x, y = 1 1
None
Ques 17. Two numbers are given by user. write a program to find different of these numbers, if first number is greater than second number otherwise find sum of numbers.
#include <stdio.h>
main()
{
int first, second;
printf("Enter two numbers = ");
scanf("%d%d", &first, &second);
if (first > second)
{
printf("Difference is %d", first - second);
}
else
{
printf("Sum is %d", first + second);
}
}
Enter two numbers = 25 11
Difference is 14
// OR
Enter two numbers = 5 6
Sum is 11
Ques 18. Three angles of a triangle are given by user . Write a program to check weather of triangle is valid or not.
#include <stdio.h>
main()
{
int a, b, c;
printf("Enter three sides of trianlge = ");
scanf("%d%d%d", &a, &b, &c);
if ((a + b + c == 180) && a != 0 && b != 0 && c != 0)
printf("Triangle is valid");
else
printf("Triangle is not valid");
}
Enter three sides of trianlge = 40 60 80
Triangle is valid
// OR
Enter three sides of trianlge = 50 70 40
Triangle is not valid
Ques 19. Three sides of triangle are given by user . Write a program to check weather the triangle is equilateral , isosceles or scalene.
#include <stdio.h>
main()
{
int a, b, c;
printf("Enter three sides of triangle = ");
scanf("%d%d%d", &a, &b, &c);
if (a == b && b == c)
printf("Triangle is Equilateral");
else if (a == b || b == c || c == a)
printf("Triangle is Isosceles");
else
printf("Triangle is Scalene");
}
Enter three sides of triangle = 30 30 30
Triangle is Equilateral
// OR
Enter three sides of triangle = 30 30 20
Triangle is Isosceles
// OR
Enter three sides of triangle = 30 20 10
Triangle is Scalene
Ques 20. Three sides of a triangle are given by the user . Write a program to check whether the triangle is valid or not.
#include <stdio.h>
main()
{
int a, b, c;
printf("Enter three sides of triangle = ");
scanf("%d%d%d", &a, &b, &c);
if (a > b && a > c)
{
if (b + c > a)
printf("Triangle is valid.");
else
printf("Triangle is not valid.");
}
else if (b > a && b > c)
{
if (a + c > b)
printf("Triangle is valid.");
else
printf("Triangle is not valid.");
}
else
{
if (a + b > c)
printf("Triangle is valid.");
else
printf("Triangle is not valid.");
}
}
Enter three sides of triangle = 20 40 35
Triangle is valid.
// OR
Enter three sides of triangle = 25 50 10
Triangle is not valid.
Ques 21. Cost price and selling price of an item are given by user. Write a program to check whether the seller has made profit , loss or no profit loss.
#include <stdio.h>
main()
{
int CP, SP;
printf("Enter cost price of item = ");
scanf("%d", &CP);
printf("Enter selling price of item = ");
scanf("%d", &SP);
// CP < SP ? printf("Seller has made profit.") : (CP > SP ? printf("Seller has made loss") : printf("Seller has made no loss."));
if (CP < SP)
printf("Seller has made profit.");
else if (CP > SP)
printf("Seller has made loss.");
else
printf("Seller has made no profit or loss.");
}
Enter cost price of item = 10
Enter selling price of item = 20
Seller has made profite.
// OR
Enter cost price of item = 20
Enter selling price of item = 10
Seller has made loss.
// OR
Enter cost price of item = 20
Enter selling price of item = 20
Seller has made no profit or loss.
Ques 22. Coordinates (x,y) of center of a circle and radius are given by the user . Write a program to check whether a given point (x1,y1) lies inside the circle , outside the circle or on the circle.
#include <stdio.h>
#include <math.h>
main()
{
float x, y, x1, y1, R, D;
// Coorditnates of center of circle = a1,b1 , radius of circle = R
D= Distance between points (x1,y1) and center
printf("Enter coordinates of center of circle = ");
scanf("%f%f", &x, &y);
printf("Enter radius of circle = ");
scanf("%f", &R);
printf("Enter coordinates of point (x1,y1) = ");
scanf("%f%f", &x1, &y1);
// Check distance between points (x1,y1) and center of circle
D = sqrt(pow((x1 - x), 2) + pow((y1 - y), 2)); // Use Distance Formula
printf("Distance between points (x1,y1) and center of circle = %f\n", D);
if (D > R)
printf("Points (%f,%f) lies outside the circle.", x1, y1);
else if (D < R)
printf("Points (%f,%f) lies inside the circle.", x1, y1);
else
printf("Points (%f,%f) lies on the circle.", x1, y1);
}
Enter coordinates of center of circle = 5 6
Enter radius of circle = 10
Enter coordinates of point (x1,y1) = 5 4
Distance between points (x1,y1) and center of circle = 2.000000
Points (5.000000,4.000000) lies inside the circle.
// OR
Enter coordinates of center of circle = 6 1
Enter radius of circle = 2
Enter coordinates of point (x1,y1) = 1 1
Distance between points (x1,y1) and center of circle = 5.000000
Points (1.000000,1.000000) lies outside the circle.
Ques 23. Marks of five subjects of a student are given by the user. Write a program to find division of the student . Division is given according to the following criteria:
Percentage>=60 – First Division
50<=Percentage<60 – Second Division
40<=Percentage<50 – Third Division
Percentage<=40 – Fail
#include <stdio.h>
main()
{
int m1, m2, m3, m4, m5, Per; // Per = Percentage
printf("Enter marks of five subjects = ");
scanf("%d%d%d%d%d", &m1, &m2, &m3, &m4, &m5);
Per = (m1 + m2 + m3 + m4 + m5) * 100 / 500;
printf("%d%%\n", Per);
if (Per >= 60)
printf("First Division");
else
{
// if (Per >= 50 && Per < 60)
if (Per >= 50)
printf("Second Division");
// else if (Per >= 40 && Per < 50)
else if (Per >= 40)
printf("Third Division");
else
printf("Fail");
}
}
Enter marks of five subjects = 60 50 70 75 55
62%
First Division
// OR
Enter marks of five subjects = 50 55 60 48 52
53%
Second Division
// OR
Enter marks of five subjects = 45 50 30 65 55
49%
Third Division
// OR
Enter marks of five subjects = 25 30 45 35 40
35%
Fail
Ques 24. Write a program to print day name using switch . Day number is given by the user . Assume that day 1 is Monday.
#include <stdio.h>
main()
{
int day;
printf("Enter day number = ");
scanf("%d", &day);
switch (day)
{
case 1:
printf("Monday");
break;
case 2:
printf("Tuesday");
break;
case 3:
printf("Wednesday");
break;
case 4:
printf("Thursday");
break;
case 5:
printf("Friday");
break;
case 6:
printf("Saturday");
break;
case 7:
printf("Sunday");
break;
default:
printf("Please enter values betweeen 1 o 7 only");
}
}
Enter day number = 3
Wednesday
// OR
Enter day number = 5
Friday
Ques 25. Write a program to find values of f. Values of a,b,c and x are given by the user. values of f is defined as follows:
f= { ax³+bx²+c ,if x=1
f= { ax²+bx+c ,if x=2
f= { ax+b+c ,if x=3
f= { ax+b ,if x=4
#include <stdio.h>
#include <math.h>
main()
{
int a, b, c, x, f;
printf("Enter values of a,b,c = ");
scanf("%d%d%d", &a, &b, &c);
printf("Enter value of x = ");
scanf("%d", &x);
switch (x)
{
case 1:
f = (a * pow(x, 3)) + (b * pow(x, 2)) + c;
printf("Value of f = %d", f);
break;
case 2:
f = (a * pow(x, 2)) + (b * x) + c;
printf("Value of f = %d", f);
break;
case 3:
f = (a * x) + b + c;
printf("Value of f = %d", f);
break;
case 4:
f = (a * x) + b;
printf("Value of f = %d", f);
break;
default:
printf("Please enter correct value of x between 1 to 4");
}
}
Enter values of a,b,c = 1 2 3
Enter value of x = 1
Value of f = 6
// OR
Enter values of a,b,c = 5 6 8
Enter value of x = 2
Value of f = 40
// OR
Enter values of a,b,c = 8 7 1
Enter value of x = 3
Value of f = 32
// OR
Enter values of a,b,c = 5 3 6
Enter value of x = 4
Value of f = 23
// OR
Enter values of a,b,c = 7 9 1
Enter value of x = 5
Please enter correct value of x between 1 to 4
Ques 26. Write a program to check whether given alphabet is Vowel or Consonant using switch statement.
#include <stdio.h>
main()
{
char alpha;
printf("Enter alphabet = ");
scanf("%c", &alpha);
switch (alpha)
{
case 'a':
case 'e':
case 'i':
case 'o':
case 'u':
case 'A':
case 'E':
case 'I':
case 'O':
case 'U':
printf("It is vowel");
break;
default:
printf("It is Consonant");
}
}
Enter alphabet = a
It is vowel
// OR
Enter alphabet = b
It is Consonant
// OR
Enter alphabet = U
It is vowel
Ques 27. Write a program to check whether roots of a quadratic equation are real and distinct , real and equal or imaginary using switch statement.
#include <stdio.h>
main()
{
int a, b, c, D;
printf("Enter values of a,b,c = ");
scanf("%d%d%d", &a, &b, &c);
D = b * b - 4 * a * c;
printf("Discriminant = %d\n", D);
switch (D > 0)
{
case 1:
printf("Roots are real and distinct");
break;
case 0:
switch (D < 0)
{
case 1:
printf("Roots are imaginary");
break;
case 0:
printf("Roots are real and equal");
break;
}
}
}
Enter values of a,b,c = 15 20 5
Discriminant = 100
Roots are real and distinct
// OR
Enter values of a,b,c = 5 10 15
Discriminant = -200
Roots are imaginary
// OR
Enter values of a,b,c = 0 0 0
Discriminant = 0
Roots are real and equal
Ques 28. Month number is given by the user . Write a program to print month name using switch statement.
#include <stdio.h>
main()
{
int month;
printf("Enter month number = ");
scanf("%d", &month);
switch (month)
{
case 1:
printf("January");
break;
case 2:
printf("Febrary");
break;
case 3:
printf("March");
break;
case 4:
printf("April");
break;
case 5:
printf("May");
break;
case 6:
printf("June");
break;
case 7:
printf("July");
break;
case 8:
printf("August");
break;
case 9:
printf("September");
break;
case 10:
printf("October");
break;
case 11:
printf("November");
break;
case 12:
printf("December");
break;
default:
printf("Please enter numbers between 1 to 12");
}
}
Enter month number = 3
March
// OR
Enter month number = 12
December
// OR
Enter month number = 15
Please enter numbers between 1 to 12
Ques 29. Write program to print this series using while loop.
10 9 8 7 6 5 4
#include <stdio.h>
main()
{
int i = 10;
while (i >= 4)
{
printf("%d ", i);
i--;
}
}
10 9 8 7 6 5 4
Ques 30. Write program to print this series using while loop.
1 3 5 7 9 11 13
#include <stdio.h>
main()
{
int i = 1;
while (i <= 13)
{
printf("%d ", i);
i = i + 2;
}
}
1 3 5 7 9 11 13
Ques 31. Write program to print this series using while loop.
2 6 10 14 18 22
#include <stdio.h>
main()
{
int i = 2;
while (i <= 22)
{
printf("%d ", i);
i = i + 4;
}
}
2 6 10 14 18 22
Ques 32. Write program to print this series using while loop.
0 5 10 15 20 25 30
#include <stdio.h>
main()
{
int i = 0;
while (i <= 30)
{
printf("%d ", i);
i = i + 5;
}
}
0 5 10 15 20 25 30
Ques 33. Write program to print this series using while loop.
20 10 5 2 1
#include <stdio.h>
main()
{
int i = 20;
while (i >= 1)
{
printf("%d ", i);
i = i / 2;
}
}
20 10 5 2 1
Ques 34. Write program to print this series using while loop.
1 2 4 7 11 16 22
#include <stdio.h>
main()
{
int i = 1, x = 1;
while (i <= 22)
{
printf("%d ", i);
i = i + x;
x++;
}
}
1 2 4 7 11 16 22
Ques 35. Write a program to find sum of all the numbers from 1 to 100 using while loop.
#include <stdio.h>
main()
{
int i = 1, sum = 0;
while (i <= 100)
{
sum = sum + i;
i++;
}
printf("Sum = %d", sum);
}
Sum = 5050
Ques 36. Write a program to find sum of all even and odd numbers between 1 and 100.
#include <stdio.h>
main()
{
int i = 1, sum = 0, sum1 = 0;
while (i <= 100)
{
if (i % 2 == 0)
{
sum = sum + i;
}
else
{
sum1 = sum1 + i;
}
i++;
}
printf("Sum of all even numbers = %d\nSum of all odd numbers = %d", sum, sum1);
}
Sum of all even numbers = 2550
Sum of all odd numbers = 2500
Ques 37. Write a program to find factorial of given numbers.
#include <stdio.h>
main()
{
int i, n, f;
printf("Enter Number = ");
scanf("%d", &n);
i = 1, f = 1;
while (i <= n)
{
f = f * i;
i++;
}
printf("Factorial of all digits = %d", f);
}
Enter Number = 5
Factorial of all digits = 120
Ques 38. Write a program to find sum of all digits of a given numbers.
#include <stdio.h>
main()
{
int n, d, sum = 0;
printf("Enter Number = ");
scanf("%d", &n);
while (n != 0)
{
d = n % 10;
sum = sum + d;
n = n / 10;
}
printf("Sum of all digits = %d", sum);
}
Enter Number = 123
Sum of all digits = 6
Ques 39. Write a program to find number of all digits given by the user.
#include <stdio.h>
main()
{
int n, count = 0;
printf("Enter Number = ");
scanf("%d", &n);
while (n != 0)
{
// count = count + 1;
count++;
n = n / 10;
}
printf("Total numbers of all digits = %d", count);
}
Enter Number = 1234
Total numbers of all digits = 4
Ques 40. Write a program to find reverse number of digits given by user.
#include <stdio.h>
main()
{
int n, d, r = 0;
printf("Enter number = ");
scanf("%d", &n);
while (n != 0)
{
d = n % 10;
r = r * 10 + d;
n = n / 10;
}
printf("Reverse of all digits = %d", r);
}
Enter number = 258
Reverse of all digits = 852
Ques 41. Write a program to check whether given number is palindrome or not.
#include <stdio.h>
main()
{
int n, d, r = 0, n1;
printf("Enter Number = ");
scanf("%d", &n);
n1 = n;
while (n != 0)
{
d = n % 10;
r = r * 10 + d;
n = n / 10;
}
if (r == n1)
{
printf("Number is palidrome.");
}
else
{
printf("Number is not palidrome.");
}
}
Enter Number = 121
Number is palindrome.
// OR
Enter Number = 456
Number is not palindrome.
Ques 42. Write a program to check whether given number is Armstrong or not.
#include <stdio.h>
main()
{
int n, d, sum = 0, n1;
printf("Enter Number = ");
scanf("%d", &n);
n1 = n;
while (n != 0)
{
d = n % 10;
sum = sum + d * d * d;
n = n / 10;
}
if (sum == n1)
{
printf("Number is armstrong.");
}
else
{
printf("Number is not armstrong.");
}
}
Enter Number = 153
Number is armstrong.
// OR
Enter Number = 567
Number is not armstrong.
Ques 43. Write a program to print the following pattern.
2 4 6 8 10 12 14 16
#include <stdio.h>
main()
{
int i;
for (i = 2; i <= 16; i = i + 2)
{
printf("%d ", i);
}
}
2 4 6 8 10 12 14 16
Ques 44. Write a program to print the following pattern.
5 10 20 40 80 160
#include <stdio.h>
main()
{
int i;
for (i = 5; i <= 160; i = i * 2)
{
printf("%d ", i);
}
}
5 10 20 40 80 160
Ques 45. Write a program to print the following pattern using for loop.
100 80 60 40 20 0
#include <stdio.h>
main()
{
int i;
for (i = 100; i >= 0; i = i - 20)
{
printf("%d ", i);
}
}
100 80 60 40 20 0
Ques 46. Write a program to find reverse of given digits using goto keyword.
#include <stdio.h>
main()
{
int r = 0, n, d;
printf("Enter Number = ");
scanf("%d", &n);
rev: // rev is a LABEL for goto
d = n % 10;
r = r * 10 + d;
n = n / 10;
if (n != 0)
goto rev;
printf("Reverse = %d", r);
}
Enter Number = 456
Reverse = 654
Ques 47. Write a program to check whether given number is prime or not.
#include <stdio.h>
main()
{
int i, n, a;
printf("Enter Number = ");
scanf("%d", &n);
a = 0; // a is flag value
for (i = 2; i <= n / 2; i++)
{
if (n % i == 0)
a++;
}
if (a == 0)
printf("It is a prime number.");
else
printf("It is not a prime number.");
}
Enter Number = 13
It is a prime number.
// OR
Enter Number = 25
It is not a prime number.
Ques 48. Write a program to print Fibonacci Series upto n terms.
#include <stdio.h>
main()
{
int i, n, first = 0, second = 1, next;
printf("Enter number of terms = ");
scanf("%d", &n);
printf("%d %d", first, second);
for (i = 1; i <= n - 2; i++)
{
next = first + second;
printf(" %d", next);
first = second;
second = next;
}
}
Enter number of terms = 5
0 1 1 2 3
// OR
Enter number of terms = 10
0 1 1 2 3 5 8 13 21 34
Ques 49. Write a program to find HCF (Highest Common Factor) of given numbers.
#include <stdio.h>
main()
{
int a, b, i, s;
printf("Enter a and b = ");
scanf("%d%d", &a, &b);
if (a < b)
{
s = a;
}
else
{
s = b;
}
for (i = s; i >= 1; i--)
{
if (a % i == 0 && b % i == 0)
{
printf("HCF = %d", i);
break;
}
}
}
Enter a and b = 25 55
HCF = 5
// OR
Enter a and b = 12 24
HCF = 12
Ques 50. Write a program to find GCD of given two numbers.
#include <stdio.h>
main()
{
int i, n1, n2, GCD_number;
printf("Enter Numbers = ");
scanf("%d%d", &n1, &n2);
for (i = 1; i <= n1 && i <= n2; i++)
{
if (n1 % i == 0 && n2 % i == 0)
{
GCD_number = i;
}
}
printf("GCD number of %d and %d = %d", n1, n2, GCD_number);
}
Enter Numbers = 25 30
GCD number of 25 and 30 = 5
// OR
Enter Numbers = 24 36
GCD number of 24 and 36 = 12
Ques 51. Write a program to print first n odd terms of Fibonacci series . Value of n is given by user.
#include <stdio.h>
main()
{
int i, n, first, second, next;
printf("Enter Number = ");
scanf("%d", &n);
first = 0;
second = 1;
printf("Second Numbers = %d", second);
for (i = 1; i <= n - 1;)
{
next = first + second;
if (next % 2 != 0)
{
printf(" %d", next);
i++;
}
first = second;
second = next;
}
}
Enter Number = 5
Second Numbers = 1 1 3 5 13
// OR
Enter Number = 10
Second Numbers = 1 1 3 5 13 21 55 89 233 377
Ques 52. Write a program to print the following pattern.
* * * * * *
* * * * * *
* * * * * *
#include <stdio.h>
main()
{
int i, j;
for (i = 1; i < 4; i++)
{
for (j = 1; j <= 6; j++)
{
printf("* ");
}
printf("\n");
}
}
* * * * * *
* * * * * *
* * * * * *
Ques 53. Write a program to print the following pattern.
*
* *
* * *
* * * *
* * * * *
#include <stdio.h>
main()
{
int i, j;
for (i = 1; i <= 5; i++)
{
for (j = 1; j <= i; j++)
{
printf("* ");
}
printf("\n");
}
}
*
* *
* * *
* * * *
* * * * *
Ques 54. Write a program to print the following pattern.
1
1 2
1 2 3
1 2 3 4
#include <stdio.h>
main()
{
int i, j;
for (i = 1; i <= 4; i++)
{
for (j = 1; j <= i; j++)
{
printf("%d ", j);
}
printf("\n");
}
}
1
1 2
1 2 3
1 2 3 4
Ques 55. Write a program to print the following pattern.
A
A B
A B C
A B C D
#include <stdio.h>
main()
{
int i, j;
char x;
for (i = 1; i <= 4; i++)
{
x = 'A';
for (j = 1; j <= i; j++)
{
printf("%c ", x);
x++;
}
printf("\n");
}
}
A
A B
A B C
A B C D
Ques 56. Write a program to print the following pattern.
* * * *
* * *
* *
*
#include <stdio.h>
main()
{
int i, j;
for (i = 1; i <= 4; i++)
{
for (j = 4; j >= i; j--)
{
printf("* ");
}
printf("\n");
}
}
* * * *
* * *
* *
*
Ques 57. Write a program to print the following pattern.
1 2 3 4
1 2 3
1 2
1
#include <stdio.h>
main()
{
int i, j;
for (i = 1; i <= 4; i++)
{
for (j = 4; j >= i; j--)
{
printf("* ");
}
printf("\n");
}
}
1 2 3 4
1 2 3
1 2
1
Ques 58. Write a program to print the following pattern.
4
4 3
4 3 2
4 3 2 1
#include <stdio.h>
main()
{
int i, j, x;
for (i = 1; i <= 4; i++)
{
x = 4;
for (j = 1; j <= i; j++)
{
printf("%d ", x);
x--;
}
printf("\n");
}
}
4
4 3
4 3 2
4 3 2 1
Ques 59. Write a program to print the following pattern.
A B C D
A B C
A B
A
#include <stdio.h>
main()
{
int i, j;
char x;
for (i = 1; i <= 4; i++)
{
x = 'A';
for (j = 4; j >= i; j--)
{
printf("%c ", x);
x++;
}
printf("\n");
}
}
A B C D
A B C
A B
A
Ques 60. Write a program to print the following pattern.
*
* *
* * *
* * * *
* * * * *
#include <stdio.h>
main()
{
int i, j, k;
for (i = 1; i <= 5; i++)
{
for (j = 4; j >= i; j--)
{
printf(" ");
}
for (k = 1; k <= i; k++)
{
printf("* ");
}
printf("\n");
}
}
*
* *
* * *
* * * *
* * * * *
Ques 61. Write a program to print the following pattern.
* * * * * * *
* * * * *
* * *
*
#include <stdio.h>
main()
{
int i, j, k;
for (i = 1; i <= 4; i++)
{
for (j = 1; j < i; j++)
{
printf(" ");
}
for (k = 7; k >= 2 * i - 1; k--)
{
printf("* ");
}
printf("\n");
}
}
* * * * * * *
* * * * *
* * *
*
Ques 62. Write a program to print the following pattern.
*
* * *
* * * * *
* * * * * * *
#include <stdio.h>
main()
{
int i, j, k;
for (i = 1; i <= 4; i++)
{
for (j = 3; j >= i; j--)
{
printf(" ");
}
for (k = 1; k <= 2 * i - 1; k++)
{
printf("* ");
}
printf("\n");
}
}
*
* * *
* * * * *
* * * * * * *
Ques 63. Write a program to print the following pattern.
A
A A
A A A
A A A A
#include <stdio.h>
main()
{
int i, j;
char x;
x = 'A';
for (i = 1; i <= 4; i++)
{
for (j = 1; j <= i; j++)
{
printf("%c ", x);
}
x++;
printf("\n");
}
}
A
A A
A A A
A A A A
Ques 64. Write a program to print the following pattern.
A
B B
C C C
D D D D
#include <stdio.h>
main()
{
int i, j;
char x;
x = 'A';
for (i = 1; i <= 4; i++)
{
for (j = 1; j <= i; j++)
{
printf("%c ", x);
}
x++;
printf("\n");
}
}
A
B B
C C C
D D D D
Ques 65. Write a program to print the following pattern.
A
A B
A B C
A B C D
#include <stdio.h>
main()
{
int i, j;
char x;
for (i = 1; i <= 4; i++)
{
x = 'A';
for (j = 1; j <= i; j++)
{
printf("%c ", x);
x++;
}
printf("\n");
}
}
A
A B
A B C
A B C D
Ques 66. Write a program to print the following pattern.
1
2 3
4 5 6
7 8 9 10
#include <stdio.h>
main()
{
int i, j, x;
x = 1;
for (i = 1; i <= 4; i++)
{
for (j = 1; j <= i; j++)
{
printf("%d ", x);
x++;
}
printf("\n");
}
}
1
2 3
4 5 6
7 8 9 10
Ques 67. Write a program to print the following pattern.
1
2 2
3 3 3
4 4 4 4
#include <stdio.h>
main()
{
int i, j, x;
x = 1;
for (i = 1; i <= 4; i++)
{
for (j = 1; j <= i; j++)
{
printf("%d ", x);
}
x++;
printf("\n");
}
}
1
2 2
3 3 3
4 4 4 4
Ques 68. Write a program to find average of 10 numbers given by user.
#include <stdio.h>
main()
{
int a[10], i, s = 0;
float avg;
printf("Enter 10 Numbers = ");
for (i = 0; i < 10; i++)
{
scanf("%d", &a[i]);
s = s + a[i];
}
avg = s / 10.0;
printf("Average of 10 Numbers = %f", avg);
}
Enter 10 Numbers = 1 2 3 4 5 6 7 8 9 10
Average of 10 Numbers = 5.500000
Ques 69. Write a program to find the largest of given n numbers.
#include <stdio.h>
main()
{
int i, n, max;
printf("Enter size of array = ");
scanf("%d", &n);
int a[n];
printf("Enter all numbers = ");
for (i = 0; i < n; i++)
{
scanf("%d", &a[i]);
}
max = a[0];
for (i = 1; i < n; i++)
{
if (max < a[i])
max = a[i];
}
printf("Largest number = %d", max);
}
Enter size of array = 5
Enter all numbers = 1 2 3 4 5
Largest number = 5
Ques 70. Write a program to find the largest of given n numbers and its location.
#include <stdio.h>
main()
{
int i, n, max, loc; // loc = location , max = maximum value
printf("Enter size of array = ");
scanf("%d", &n);
int a[n];
printf("Enter all numbers = ");
for (i = 0; i < n; i++)
{
scanf("%d", &a[i]);
}
max = a[0];
loc = 0;
for (i = 1; i < n; i++)
{
if (max < a[i])
{
loc = i;
max = a[i];
}
}
printf("Largest number is %d and location is %d", max, loc);
}
Enter size of array = 5
Enter all numbers = 1 2 3 4 5
Largest number is 5 and location is 4
Ques 71. Write a program to reverse an array.
#include <stdio.h>
void main()
{
int i, n, c, a[500];
printf("Enter size of array = ");
scanf("%d", &n);
printf("Enter all numbers of array = ");
for (i = 0; i < n; i++)
{
scanf("%d", &a[i]);
}
for (i = 0; i < n / 2; i++)
{
c = a[i];
a[i] = a[n - 1 - i];
a[n - 1 - i] = c;
}
printf("Reverse array = ");
for (i = 0; i < n; i++)
{
printf("%d ", a[i]);
}
}
Enter size of array = 5
Enter all numbers of array = 1 2 3 4 5
Reverse array = 5 4 3 2 1
Ques 72. Write a program to short an array.
#include <stdio.h>
void main()
{
int i, n, p, c, a[500]; // n = array size , p = 1 complete pass
printf("Enter size of array = ");
scanf("%d", &n);
printf("Enter all numbers of array = ");
for (i = 0; i < n; i++)
{
scanf("%d", &a[i]);
}
for (p = 1; p <= n - 1; p++)
{
for (i = 0; i <= n - 1 - p; i++)
{
if (a[i] > a[i + 1])
{
c = a[i];
a[i] = a[i + 1];
a[i + 1] = c;
}
}
}
printf("Shorted array = ");
for (i = 0; i < n; i++)
{
printf("%d ", a[i]);
}
}
Enter size of array = 5
Enter all numbers of array = 2 8 1 6 9
Shorted array = 1 2 6 8 9
Ques 73. Write a program to find location of element in array
#include <stdio.h>
main()
{
int a[500], i, n, beg, end, mid, item;
printf("Enter size of array = ");
scanf("%d", &n);
printf("Enter all elements = ");
for (i = 0; i < n; i++)
{
scanf("%d", &a[i]);
}
printf("Enter the element to be searched = ");
scanf("%d", &item);
beg = 0;
end = n - 1;
mid = (beg + end) / 2;
while (item != a[mid] && beg <= end)
{
if (item > a[mid])
{
beg = mid + 1;
}
else
{
end = mid - 1;
}
mid = (beg + end) / 2;
}
if (item == a[mid])
printf("location = %d", mid);
else
printf("item not found.");
}
Enter size of array = 5
Enter all elements = 1 2 3 4 5
Enter the element to be searched = 4
location = 3
Ques 74. Write a program to print 2X4 matrix Two-D array.
#include <stdio.h>
main()
{
int a[2][4], i, j;
printf("Enter matrix of 2x4 = \n");
for (i = 0; i < 2; i++)
{
for (j = 0; j < 4; j++)
{
scanf("%d", &a[i][j]);
}
}
printf("Matrix is \n");
for (i = 0; i < 2; i++)
{
for (j = 0; j < 4; j++)
{
printf("%d ", a[i][j]);
}
printf("\n");
}
}
Enter matrix of 2x4 =
1 2 3 4
5 6 7 8
Matrix is
1 2 3 4
5 6 7 8
Ques 75. Write a program to sum all elements of 2X4 matrix Two-D array.
#include <stdio.h>
main()
{
int a[2][4], i, j, sum = 0;
printf("Enter matrix of 2x4 = \n");
for (i = 0; i < 2; i++)
{
for (j = 0; j < 4; j++)
{
scanf("%d", &a[i][j]);
}
}
printf("Matrix Sum is \n");
for (i = 0; i < 2; i++)
{
for (j = 0; j < 4; j++)
{
sum = sum + a[i][j];
}
}
printf("%d",sum);
}
Enter matrix of 2x4 =
1 2 3 4
5 6 7 8
Matrix Sum is
36
Ques 76. Write a program to find largest element in Two Dimensional array.
#include <stdio.h>
main()
{
int i, j, max, r, c;
printf("Enter order of matrix = ");
scanf("%d%d", &r, &c);
int a[r][c];
printf("Enter matrix = \n");
for (i = 0; i < r; i++)
{
for (j = 0; j < c; j++)
{
scanf("%d", &a[i][j]);
}
}
max = a[0][0];
for (i = 0; i < r; i++)
{
for (j = 0; j < c; j++)
{
if (max < a[i][j])
max = a[i][j];
}
}
printf("Largest element = %d", max);
}
Enter order of matrix = 2 3
Enter matrix =
1 2 3
4 5 6
Largest element = 6
Ques 77. Write a program to find diagonal elements of Two Dimensional array.
#include <stdio.h>
main()
{
int i, j, r, c;
printf("Enter order of matrix = ");
scanf("%d%d", &r, &c);
int a[r][c];
printf("Enter matrix = \n");
for (i = 0; i < r; i++)
{
for (j = 0; j < c; j++)
{
scanf("%d", &a[i][j]);
}
}
printf("Diagonal element of array \n");
for (i = 0; i < r; i++)
{
for (j = 0; j < c; j++)
{
if (i == j)
printf("%d \n", a[i][j]);
}
}
}
Enter order of matrix = 4 4
Enter matrix =
1 2 3 4
5 6 7 8
4 3 2 1
8 7 6 5
Diagonal element of array
1
6
2
5
Ques 78. Write a program to print string and string is given by user.
#include <stdio.h>
main()
{
char st[5];
printf("Enter string = ");
gets(st);
printf("Entered string = ");
puts(st);
}
Enter string = hello
Entered string = hello
Ques 79. Write a program to check whether given string is palindrome or not.
#include <stdio.h>
#include <string.h>
main()
{
char st1[50], st2[50];
printf("Enter any string = ");
gets(st1);
strcpy(st2, st1);
strrev(st1);
if (strcmp(st1, st2) == 0)
printf("It is palindrome");
else
printf("It is not palindrome");
}
Enter any string = dad
It is palindrome
//OR
Enter any string = Shubham
It is not palindrome
Ques 80. Write a program to create record of two students using structure data type.
#include <stdio.h>
struct student
{
int rollno;
char name[50];
float per;
};
main()
{
struct student a, b;
printf("Enter details of first student = ");
scanf("%d%s%f", &a.rollno, a.name, &a.per);
printf("Enter details of second student = ");
scanf("%d%s%f", &b.rollno, b.name, &b.per);
printf("Record of students is \n");
printf("%d %s %f\n", a.rollno, a.name, a.per);
printf("%d %s %f", b.rollno, b.name, b.per);
}
Enter details of first student = 1 shubham 85
Enter details of second student = 2 rahul 60
Record of students is
1 shubham 85.000000
2 rahul 60.000000
Ques 81. Write a program to create record of employees of an organization having members employee name , age , experience and salary . Then print the record of all the employees having experience more than 10 years. Assume that there are 5 employees in the organization.
#include <stdio.h>
struct employee
{
int age, salary;
char name[5];
float experience;
};
main()
{
int i;
struct employee a[5];
printf("Enter all details of employees ");
for (i = 0; i < 5; i++)
{
scanf("%d%d%s%f", &a[i].age, &a[i].salary, a[i].name, &a[i].experience);
}
printf("Record is \n");
for (i = 0; i < 5; i++)
{
if (a[i].experience > 10)
printf("%d %d %s %f\n", a[i].age, a[i].salary, a[i].name, a[i].experience);
}
}
Enter all details of employees
20 10000 shubham 1
22 15000 rahul 11
21 18000 jay 12
23 16000 mahesh 5
24 17000 vikas 8
Record is
22 15000 rahul 11.000000
21 18000 jay 12.000000
Ques 82. Write a program to create record of students of a class having fields student roll number, name and percentage. Than print record of the student whose name is given by user.
#include <stdio.h>
struct student
{
int rollno;
char name[50];
float per;
};
main()
{
int i;
char st[50];
struct student a[5];
printf("Enter details of all student \n");
for (i = 0; i < 5; i++)
{
scanf("%d%s%f", &a[i].rollno, a[i].name, &a[i].per);
}
printf("Enter name of student : ");
scanf("%s", st);
printf("Record is \n");
for (i = 0; i < 5; i++)
{
if (strcmp(st, a[i].name)==0)
printf("%d %s %f \n", a[i].rollno, a[i].name, a[i].per);
}
}
Enter details of all student
1 shubham 60
2 raj 56
3 arun 85
4 suresh 80
5 raju 75
Enter name of student : arun
Record is
3 arun 85.000000
Ques 83. Write a program to create record of students of a class having fields student roll number, name and percentage. Than print record of the student whose percentage is greater than 80.
#include <stdio.h>
struct student
{
int rollno;
char name[50];
float per;
};
main()
{
int i;
struct student a[5];
printf("Enter details of all student \n");
for (i = 0; i < 5; i++)
{
scanf("%d%s%f", &a[i].rollno, a[i].name, &a[i].per);
}
printf("Record is \n");
for (i = 0; i < 5; i++)
{
if (a[i].per > 80)
printf("%d %s %f \n", a[i].rollno, a[i].name, a[i].per);
}
}
Enter details of all student
1 rahul 75
2 ramesh 89
3 raj 82
4 umesh 60
5 kamal 71
Record is
2 ramesh 89.000000
3 raj 82.000000
Ques 84. Write a program to create record of students of a class having fields student roll number, name and percentage. Than print record of students in increasing order of students percentage.
#include <stdio.h>
struct student
{
int rollno;
char name[50];
float per;
};
main()
{
int i, pass;
struct student a[5], c;
printf("Enter details of all student \n");
for (i = 0; i < 5; i++)
{
scanf("%d%s%f", &a[i].rollno, a[i].name, &a[i].per);
}
for (pass = 1; pass <= 5 - 1; pass++)
{
for (i = 0; i <= 5 - 1 - pass; i++)
{
if (a[i].per > a[i + 1].per)
{
c = a[i];
a[i] = a[i + 1];
a[i + 1] = c;
}
}
}
printf("Record of students in increasing order of their percentage \n");
for (i = 0; i < 5; i++)
{
printf("%d %s %f \n", a[i].rollno, a[i].name, a[i].per);
}
}
Enter details of all student
1 ram 56
2 shyam 82
3 umesh 60
4 karan 89
5 rakesh 71
Record of students in increasing order of their percentage
1 ram 56.000000
3 umesh 60.000000
5 rakesh 71.000000
2 shyam 82.000000
4 karan 89.000000
#include <stdio.h>
struct books
{
int edition;
char name[50], author[50], publisher[50];
float price;
};
main()
{
int i, pass;
struct books a[5], c;
printf("Enter details of all books \n");
for (i = 0; i < 5; i++)
{
scanf("%d%s%s%s%f", &a[i].edition, a[i].name, a[i].author, a[i].publisher, &a[i].price);
}
for (pass = 1; pass <= 5 - 1; pass++)
{
for (i = 0; i <= 5 - 1 - pass; i++)
{
if (a[i].price > a[i + 1].price)
{
c = a[i];
a[i] = a[i + 1];
a[i + 1] = c;
}
}
}
printf("Record of all books in the ascending order of their price \n");
for (i = 0; i < 5; i++)
{
printf("%d %s %s %s %f \n", a[i].edition, a[i].name, a[i].author, a[i].publisher, a[i].price);
}
}
Enter details of all books
1 maths shubham pub1 500
2 english karan pub2 300
3 hindi raj pub3 600
4 physics rahul pub4 150
5 computer umesh pub5 800
Record of all books in the ascending order of their price
4 physics rahul pub4 150.000000
2 english karan pub2 300.000000
1 maths shubham pub1 500.000000
3 hindi raj pub3 600.000000
5 computer umesh pub5 800.000000
Ques 86. Write a program to print values of some given variables using enum keyword.
#include <stdio.h>
main()
{
enum value
{
a , b, c, d , e, f
};
printf("%d %d %d %d %d %d", a, b, c,d,e,f);
}
0 1 2 3 4 5
Ques 87. Write a program to print values of some given variables using enum keyword and some variables values are given.
#include <stdio.h>
main()
{
enum value
{
a = 1, b, c, d = 0, e, f
};
printf("%d %d %d %d %d %d", a, b, c,d,e,f);
}
1 2 3 0 1 2
Ques 88. Write a program to find sum of three numbers given by user using function.
#include <stdio.h>
void sum(float, float, float); // function prototype
void sum(float a, float b, float c) // function definition
{
float s;
s = a + b + c;
printf("Sum = %f", s);
}
main()
{
float a, b, c;
printf("Enter three numbers = ");
scanf("%f%f%f", &a, &b, &c);
sum(a,b,c); //function call
}
Enter three numbers = 1 2 3
Sum = 6.000000
Ques 89. Write a program to find factorial of a given number using function.
#include <stdio.h>
int fact(int x)
{
int i, f = 1;
for (i = 1; i <= x; i++)
{
f = f * i;
}
return (f);
}
main()
{
int n;
printf("Enter number = ");
scanf("%d", &n);
printf("Factorial = %d", fact(n));
}
Enter number = 5
Factorial = 120
Ques 90. Write a program to check whether given number is prime or not using function.
#include <stdio.h>
int prime(int x)
{
int i, a = 0;
for (i = 2; i <= x / 2; i++)
{
if (x % i == 0)
a++;
break;
}
if (a == 0)
{
printf("Number is prime.");
}
else
{
printf("Number is not prime.");
}
}
main()
{
int n, p;
printf("Enter Number = ");
scanf("%d", &n);
prime(n);
}
Enter Number = 31
Number is prime.
// OR
Enter Number = 12
Number is not prime.
Ques 91. Write a user defined function to find average of three numbers.
#include <stdio.h>
int average(int a, int b, int c)
{
int s;
s = (a + b + c) / 3;
return (s);
}
main()
{
int x, y, z;
printf("Enter three numbers = ");
scanf("%d%d%d", &x, &y, &z);
printf("Average of three numbers = %d", average(x, y, z));
}
Enter three numbers = 1 2 3
Average of three numbers = 2
// OR
Enter three numbers = 5 10 15
Average of three numbers = 10
Ques 92. Write a program to copy an array.
#include <stdio.h>
main()
{
int i, n, a[500], b[500];
printf("Enter array size = ");
scanf("%d", &n);
printf("Enter all elements of array = ");
for (i = 0; i < n; i++)
{
scanf("%d", &a[i]);
b[i] = a[i];
}
printf("Copied Array = ");
for (i =0; i < n; i++)
{
printf("%d ", a[i]);
}
}
Enter array size = 5
Enter all elements of array = 1 2 3 4 5
Copied Array = 1 2 3 4 5
Ques 93. Write a program to find factorial of a number given by user using function.
#include <stdio.h>
int fact(int); //prototype
int main()
{
int n, f;
printf("Enter number = ");
scanf("%d", &n);
f = fact(n); //call
printf("Factorial = %d", f);
}
int fact(int x) //definition
{
int f = 1, i;
for (i = 1; i <= x; i++)
f = f * i;
return f;
}
Enter number = 6
Factorial = 720
Ques 94. Write a program to swap to numbers given by user using pointer.
#include <stdio.h>
void swap(int *, int *);
main()
{
int a, b;
printf("Enter two numbers = ");
scanf("%d%d", &a, &b);
swap(&a, &b);
printf("After swapping a = %d , b = %d ", a, b);
}
void swap(int *x, int *y)
{
*x = *x + *y;
*y = *x - *y;
*x = *x - *y;
}
Enter two numbers = 1 2
After swapping a = 2 , b = 1
Ques 95. Write a program to find area and perimeter of rectangle using user defined function and user defined function should return the values of area and perimeter to the main function.
#include <stdio.h>
void rect(int, int, int *, int *);
main()
{
int l, b, area, peri;
printf("Enter sides of rectangle = ");
scanf("%d%d", &l, &b);
rect(l, b, &area, &peri);
printf("Area = %d and Perimeter = %d", area, peri);
}
void rect(int a, int b, int *c, int *d)
{
*c = a * b;
*d = 2 * (a + b);
}
Enter sides of rectangle = 10 20
Area = 200 and Perimeter = 60
Ques 96. Write a function with return type and with parameter to find sum of two numbers.
#include <stdio.h>
int sum(int, int); // prototype
int sum(int a, int b)
{
return (a + b);
}
main()
{
int a, b, s;
printf("Enter two values = ");
scanf("%d%d", &a, &b);
s = a + b;
printf("Sum = %d", s);
}
Enter two values = 5 6
Sum = 11
Ques 97. Write a function with return type and without parameter to find sum of two numbers.
#include <stdio.h>
int sum(); // prototype
int sum()
{
int a, b;
printf("Enter two numbers");
scanf("%d%d", &a, &b);
return (a + b);
}
main()
{
int a, b, s;
printf("Enter two values = ");
scanf("%d%d", &a, &b);
s = a + b;
printf("Sum = %d", s);
}
Enter two values = 2 3
Sum = 5
Ques 98. Write a function without return type and with parameter to find sum of two numbers.
#include <stdio.h>
void sum(int, int); // prototype
void sum(int a, int b)
{
int s = a + b;
printf("Sum = %d", s);
}
main()
{
int a, b, s;
printf("Enter two values = ");
scanf("%d%d", &a, &b);
sum(a, b);
}
Enter two values = 9 11
Sum = 20
Ques 99. Write a function without return type and without parameter to find sum of two numbers.
#include <stdio.h>
void sum(); // prototype
void sum()
{
int a, b, s;
printf("Enter two numbers = ");
scanf("%d%d", &a, &b);
s = a + b;
printf("Sum = %d", s);
}
main()
{
sum();
}
Enter two numbers = 15 20
Sum = 35
Ques 100. Write a program to find sum of the following series.
1! + 2! + 3! + ……….. + n!
#include <stdio.h>
main()
{
int i, n, s = 0;
printf("Enter number of terms = ");
scanf("%d", &n);
for (i = 1; i <= n; i++)
{
s = s + fact(i);
}
printf("Sum of factorial numbers series = %d", s);
}
int fact(int n)
{
int f = 1, i;
for (i = 1; i <= n; i++)
{
f = f * i;
}
return f;
}
Enter number of terms = 5
Sum of factorial numbers series = 153
Ques 101. Write a program to find sum of the following series.
1 + 3^3/3! + 5^5/5! +…………………….n terms.
#include <stdio.h>
#include <math.h>
main()
{
int i, n, s = 0;
printf("Enter number of terms = ");
scanf("%d", &n);
for (i = 1; i <= n; i += 2)
{
s = s + pow(i, i) / fact(i);
}
printf("Sum of factorial numbers series = %d", s);
}
int fact(int n)
{
int f = 1, i;
for (i = 1; i <= n; i++)
{
f = f * i;
}
return f;
}
Enter number of terms = 10
Sum of factorial numbers series = 1261
Ques 102. Write a program to search an element in array using linear search.
#include <stdio.h>
main()
{
int i, n, a[500], key;
printf("Enter size of array = ");
scanf("%d", &n);
printf("Enter all elements of array = ");
for (i = 0; i < n; i++)
{
scanf("%d", &a[i]);
}
printf("Enter the element to be searched = ");
scanf("%d", &key);
for (i = 0; i < n; i++)
{
if (a[i] == key)
break;
}
if (i < n)
{
printf("Location of searched element = %d", i);
}
else
{
printf("Sorry, Element not found in this array.");
}
}
Enter size of array = 5
Enter all elements of array = 1 2 3 4 5
Enter the element to be searched = 4
Location of searched element = 3
// OR
Enter size of array = 5
Enter all elements of array = 1 2 3 4 5
Enter the element to be searched = 6
Sorry, Element not found in this array.
Ques 103. Write a program to find sum of the following series.
1+2+3+4+………………..n terms
#include <stdio.h>
int sum(int);
int sum(int n)
{
if (n != 0)
return n + sum(n - 1);
else
return 0;
}
main()
{
int n, total;
printf("Enter number of terms = ");
scanf("%d", &n);
total = sum(n);
printf("Sum of series = %d", total);
}
Enter number of terms = 5
Sum of series = 15
Ques 104. Write a program to find sum of the following series.
1+2^2+3^3+4^4+…………………n terms.
#include <stdio.h>
int sum(int);
int sum(int n)
{
int i, s = 0;
for (i = 1; i <= n; i++)
{
s = s + i * i;
}
return s;
}
main()
{
int n, total;
printf("Enter number of terms = ");
scanf("%d", &n);
total = sum(n);
printf("Sum of series = %d", total);
}
Enter number of terms = 5
Sum of series = 55
Ques 105. Write a function to find ‘a’ raise to the power ‘b’.
#include <stdio.h>
int power(int, int); // prototype
int power(int a, int b)
{
int p = 1, i;
for (i = 1; i <= b; i++)
{
p = p * a;
}
return p;
}
main()
{
int a, b;
printf("Enter values of a and b = ");
scanf("%d%d", &a, &b);
printf("'a' raise to the power 'b' = %d", power(a, b));
}
Enter values of a and b = 2 3
'a' raise to the power 'b' = 8
Ques 106. Write a program to find sum of the following series.
1-2^2/2!+3^3/3!-4^4/4!+…………………….n terms.
#include <stdio.h>
#include <math.h>
main()
{
int i, n, s;
printf("Enter number of terms = ");
scanf("%d", &n);
for (i = 1; i <= n; i++)
{
s = s + pow(-1, i + 1) * pow(i, i) / fact(i);
}
printf("Sum of given series = %d", s);
}
int fact(int n)
{
int f = 1, i;
for (i = 1; i <= n; i++)
{
f = f * i;
}
return f;
}
Enter number of terms = 5
Sum of given series = 19
Ques 107. Write a program to find factorial of given number using recursive function.
#include <stdio.h>
int fact(int); // prototype
int fact(int n)
{
if (n == 1)
return 1;
else
return n * fact(n - 1);
}
main()
{
int n;
printf("Enter Number = ");
scanf("%d", &n);
printf("Factorial = %d", fact(n));
}
Enter Number = 5
Factorial = 120
Ques 108. Write a program to find nth term of Fibonacci series using recursive function.
#include <stdio.h>
int fib(int n)
{
if (n == 1)
return 0;
else if (n == 2)
return 1;
else
return fib(n - 1) + fib(n - 2);
}
main()
{
int n, i;
printf("Enter number of terms = ");
scanf("%d", &n);
for (i = 1; i <= n; i++)
{
printf("%d ", fib(i));
}
}
Enter number of terms = 5
0 1 1 2 3
// OR
Enter number of terms = 10
0 1 1 2 3 5 8 13 21 34
Ques 109. Write a program to find sum of all digits of given number using recursion.
#include <stdio.h>
int sum(int);
int sum(int n)
{
static int s = 0;
int d;
if (n != 0)
{
d = n % 10;
s = s + d;
n = n / 10;
sum(n);
}
else
return s;
}
main()
{
int n, total;
printf("Enter Number = ");
scanf("%d", &n);
total = sum(n);
printf("Sum of all digits = %d", total);
}
Enter Number = 567
Sum of all digits = 18
Ques 110. Write a program to find GCD of two numbers using recursion.
#include <stdio.h>
int gcd(int, int);
int gcd(int n1, int n2)
{
if (n2 != 0)
return gcd(n2, n1 % n2);
else
return n1;
}
main()
{
int n, n1, n2;
printf("Enter two numbers = ");
scanf("%d%d", &n1, &n2);
printf("GCD Number of %d and %d = %d", n1, n2, gcd(n1, n2));
}
Enter two numbers = 15 25
GCD Number of 15 and 25 = 5
// OR
Enter two numbers = 25 75
GCD Number of 25 and 75 = 25
Ques 111. Write a program to find reverse of a given number using recursion.
#include <stdio.h>
int rev(int);
int rev(int n)
{
static int r = 0;
int d;
if (n != 0)
{
d = n % 10;
r = r * 10 + d;
n = n / 10;
rev(n);
}
else
return r;
}
main()
{
int n, reverse;
printf("Enter Number = ");
scanf("%d", &n);
reverse = rev(n);
printf("Reverse Number = %d", reverse);
}
Enter Number = 567
Reverse Number = 765
// OR
Enter Number = 123
Reverse Number = 321
Ques 112. Write a program to find sum of digits using recursion with pointer.
#include <stdio.h>
int sod(int *); // prototype
int sod(int *n)
{
static int s = 0;
int d;
if (*n == 0)
return s;
else
{
d = *n % 10;
s = s + d;
*n = *n / 10;
sod(n);
}
}
main()
{
int x;
printf("Enter Number = ");
scanf("%d", &x);
printf("Sum of digits = %d\n", sod(&x));
printf("After function call the value of x = %d", x);
}
Enter Number = 456
Sum of digits = 15
After function call the value of x = 0
Ques 113. Write a program to store n numbers given by user using malloc function. Then find sum of all given numbers.
#include <stdio.h>
#include <stdlib.h>
main()
{
int n, s = 0, i, *p;
printf("Enter the number of elements to be stored = ");
scanf("%d", &n);
p = (int *)malloc(n * sizeof(int));
printf("Enter all numbers = ");
for (i = 0; i <= n - 1; i++)
{
scanf("%d", p + i);
}
for (i = 0; i <= n - 1; i++)
{
s = s + *(p + i);
}
printf("Sum of all elements = %d", s);
}
Enter the number of elements to be stored = 5
Enter all numbers = 1 2 3 4 5
Sum of all elements = 15
Ques 114. Write a program to find the largest element of given n numbers stored by using malloc function.
#include <stdio.h>
#include <stdlib.h>
main()
{
int i, n, max, *p;
printf("Enter the number of elements to be stored = ");
scanf("%d", &n);
p = (int *)malloc(n * sizeof(int));
printf("Enter all numbers = ");
for (i = 0; i <= n - 1; i++)
{
scanf("%d", p + i);
}
max = 1;
for (i = 0; i <= n - 1; i++)
{
if (max < *(p + i))
max = *(p + i);
}
printf("Largest number = %d", max);
}
Enter the number of elements to be stored = 5
Enter all numbers = 4 8 1 3 6
Largest number = 8
Ques 115. Write a program to store n numbers using dynamic memory allocation then sort all the numbers in decreasing order.
#include <stdio.h>
#include <stdlib.h>
main()
{
int i, n, *p, pass, c;
printf("Enter size of numbers to be stored = ");
scanf("%d", &n);
printf("Enter all numbers = ");
for (i = 0; i <= n - 1; i++)
{
scanf("%d", (p + i));
}
for (pass = 1; pass <= n - 1; pass++)
{
for (i = 0; i <= n - 1 - pass; i++)
{
if (*(p + i) < *(p + i + 1))
{
c = *(p + i);
*(p + i) = *(p + i + 1);
*(p + i + 1) = c;
}
}
}
printf("Sort all numbers \n");
for (i = 0; i <= n - 1; i++)
{
printf("%d ", *(p + i));
}
}
Enter size of numbers to be stored = 5
Enter all numbers = 1 2 3 4 5
Sort all numbers
5 4 3 2 1
Ques 116. Write a program to find smallest number using calloc() function.
#include <stdio.h>
main()
{
int n, *p, i, min;
printf("How many numbers are there = ");
scanf("%d", &n);
p = (int *)calloc(n, sizeof(int));
printf("Enter all numbers = ");
for (i = 0; i < n; i++)
{
scanf("%d", p + i);
}
min = *p;
for (i = 1; i < n; i++)
{
if (min > *(p + i))
{
min = *(p + i);
}
}
printf("The smallest number = %d", min);
}
How many numbers are there = 5
Enter all numbers = 9 1 4 2 6
The smallest number = 1
Ques 117. Write a program to create extra memory spaces and add extra elements in array using realloc() function.
#include <stdio.h>
main()
{
int n, *p, i, n1;
char choice;
printf("How many numbers are there = ");
scanf("%d", &n);
p = (int *)malloc(n * sizeof(int));
printf("Enter all numbers = ");
for (i = 0; i < n; i++)
{
scanf("%d", p + i);
}
printf("Do you want to store more data? y or n : ");
scanf(" %c", &choice);
if (choice == 'y')
{
printf("How many additional elements are there? : ");
scanf("%d", &n1);
p = (int *)realloc(p, (n + n1) * sizeof(int));
printf("Enter all additional elements = ");
for (i = n; i < n + n1; i++)
{
scanf("%d", p + i);
}
printf("List of all new elements \n");
for (i = n; i < n + n1; i++)
{
printf("%d ", *(p + i));
}
}
free(p); // use of free() function
}
How many numbers are there = 5
Enter all numbers = 1 2 3 4 5
Do you want to store more data? y or n : y
How many additional elements are there? : 5
Enter all additional elements = 6 7 8 9 10
List of all new elements
6 7 8 9 10
Ques 118. Write a program to sort an array using Insertion Sort.
#include <stdio.h>
main()
{
int i, j, n, temp;
printf("Enter size of array = ");
scanf("%d", &n);
int a[n];
printf("Enter all numbers = ");
for (i = 0; i < n; i++)
{
scanf("%d", &a[i]);
}
for (i = 1; i < n; i++)
{
temp = a[i], j = i - 1;
while (temp < a[j] && j >= 0)
{
a[j + 1] = a[j];
j = j - 1;
}
a[j + 1] = temp;
}
printf("Sorted List \n");
for (i = 0; i < n; i++)
{
printf("%d ", a[i]);
}
}
Enter size of array = 5
Enter all numbers = 8 4 1 6 3
Sorted List
1 3 4 6 8
Ques 119. Write a program to sort an array in ascending order using selection sort.
#include <stdio.h>
main()
{
int i, j, min, index, n;
printf("Enter Size of array = ");
scanf("%d", &n);
int a[n];
printf("Enter all elements of array = ");
for (i = 0; i < n; i++)
{
scanf("%d", &a[i]);
}
for (i = 0; i <= n - 2; i++)
{
min = a[i], index = i;
for (j = i + 1; j <= n - 1; j++)
{
if (min > a[j])
{
min = a[j];
index = j;
}
}
a[index] = a[i];
a[i] = min;
}
printf("Sorted Array \n");
for (i = 0; i < n; i++)
{
printf("%d ", a[i]);
}
}
Enter Size of array = 5
Enter all elements of array = 8 1 4 6 3
Sorted Array
1 3 4 6 8
Ques 120. Write a program to print the following pattern.
*
* *
* *
* *
*********
#include <stdio.h>
main()
{
int i, j, k;
for (i = 1; i <= 4; i++)
{
for (j = 4; j >= i; j--)
{
printf(" ");
}
printf("*");
for (k = 3; k <= 2*i-1; k++)
{
printf(" ");
}
if (i >= 2)
{
printf("*");
}
printf("\n");
}
for (i = 1; i <= 9; i++)
{
printf("*");
}
}
*
* *
* *
* *
*********
निष्कर्ष – C Programs Notes
दोस्तों मैं उम्मीद करता हूँ कि मैंने इस पोस्ट के माध्यम से आपको C language program notes यानि कि जो C Language के सभी प्रोग्राम के नोट्स प्रदान किये है वे सभी नोट्स आपके लिए जरुर फायदेमंद सिद्ध हुए होंगे और जिनके माध्यम से आपको C Language के सभी प्रोग्राम्स को समझने में भी बोहोत आसानी होगी |
पोस्ट को पूरा पढने के लिए धन्यवाद ! अगर आपका इस पोस्ट से सम्बन्धित कोई भी प्रश्न है तो आप नीचे कमेंट करके पूंछ सकते है |
यह भी पढ़ें –
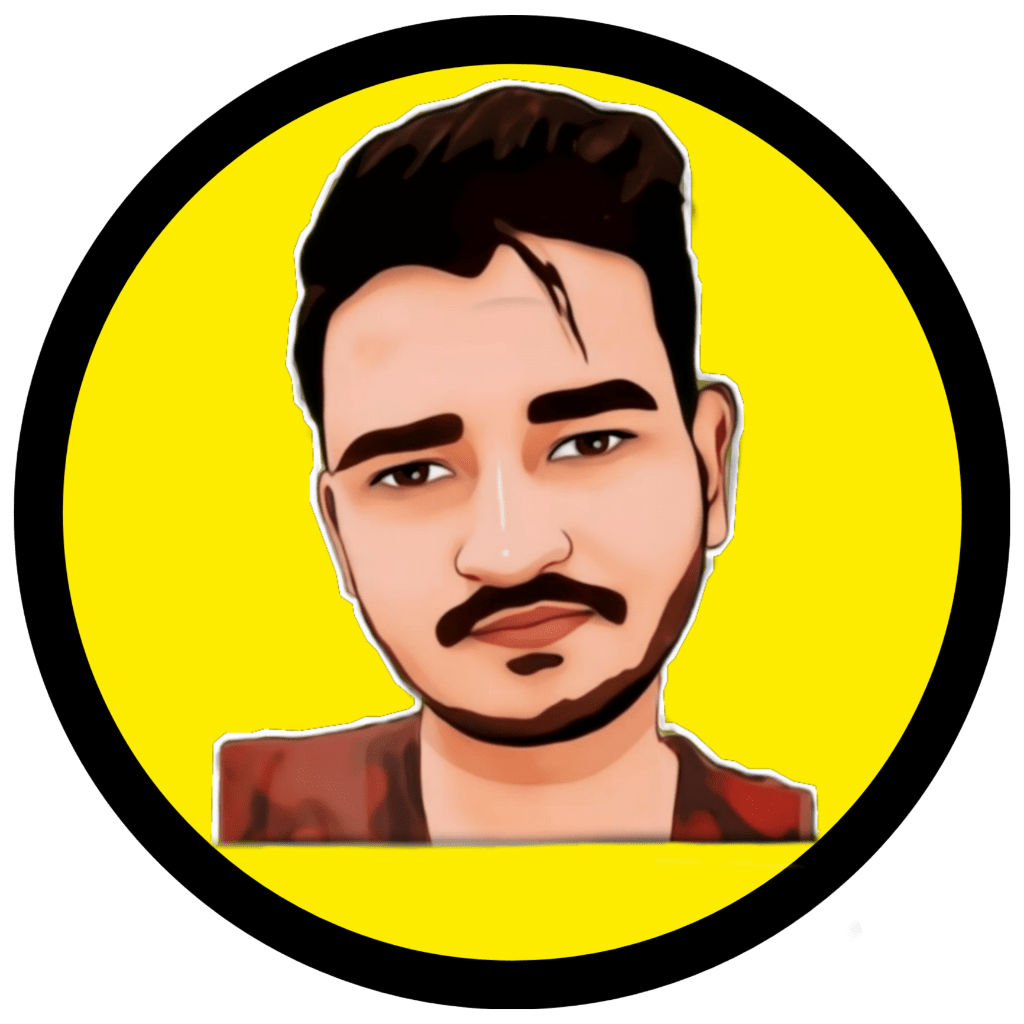
Shubham Pal (शुभम पाल) एक Digital Creator है जिसका हिन्दी ब्लॉग shubhampal.co.in है | इस ब्लॉग पर आपको टेक्नोलॉजी और कंप्यूटर से सम्बंधित बोहत सारी चीजो के बारे में बोहोत ही सरल भाषा में सीखने को मिलता है इसके साथ-साथ हमारे इस हिंदी ब्लॉग पर आपको YouTube , Blogging , Affiliate Marketing और ऑनलाइन पैसा कमाने के बोहोत सारे तरीको के बारे में भी जानने और सीखने को मिलता है |